Java Math.random() Function: Number Generation Guide
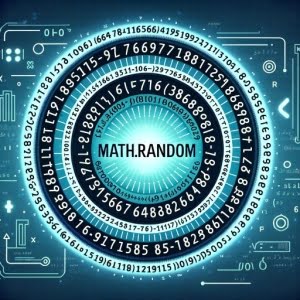
Are you looking to add a touch of unpredictability to your Java programs? Like a roll of the dice, the Math.random() function in Java can introduce randomness into your code. This function is a powerful tool that can enhance your programs in various ways, from generating random numbers for a game to selecting a random element from an array.
This guide will walk you through the ins and outs of using Math.random() to generate random numbers in Java. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches. We’ll also discuss common issues and their solutions, and provide practical examples to help you understand the concepts better.
So, let’s dive in and start mastering Math.random in Java!
TL;DR: How Do I Use Math.random() in Java?
To generate a random number in Java, you can assign the Math.random() function to variable as a value, such as,
double random = Math.random();
This function returns a double value with a positive sign, greater than or equal to 0.0 and less than 1.0.
Here’s an intermediate example:
int min = 10;
int max = 100;
int randomNum = (int)(Math.random() * (max - min + 1)) + min;
System.out.println(randomNum);
# Output:
# A random number between 10 (inclusive) and 100 (inclusive)
In this example, we’ve used Math.random()
function to generate a random integer within a specified range (from 10 to 100). We calculate the random number by multiplying the result of Math.random()
by the size of the range (max - min + 1)
, then convert the result to an integer and add the minimum value.
This is an intermediate way to use Math.random() in Java, but there’s much more to learn about generating random numbers in Java. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Generating Random Numbers in Java with Math.random()
- Generating Random Numbers Within a Range in Java
- Generating Random Integers in Java
- Exploring Alternative Methods for Random Number Generation in Java
- Troubleshooting Common Issues with Math.random() in Java
- Best Practices and Optimization
- Understanding Random Number Generation in Java
- Exploring the Impact of Random Number Generation
- Wrapping Up: Mastering Math.random() in Java
Generating Random Numbers in Java with Math.random()
Math.random() is a built-in function in Java, used to generate pseudo-random numbers. The function returns a double value with a positive sign, greater than or equal to 0.0 and less than 1.0. Here’s how you can use Math.random() to generate a random number:
double random = Math.random();
System.out.println(random);
# Output:
# A random number between 0.0 (inclusive) and 1.0 (exclusive)
In this code, Math.random()
generates a random number and assigns it to the random
variable. The System.out.println(random);
line then prints this random number to the console.
Advantages and Pitfalls of Math.random()
One of the main advantages of Math.random() is its simplicity. It’s easy to use and understand, making it a great choice for beginners. However, Math.random() only generates double values between 0.0 and 1.0. If you need to generate random integers or random numbers within a certain range, you’ll need to use a different approach, which we’ll cover in the next section.
Generating Random Numbers Within a Range in Java
While Math.random() generates a double value between 0.0 and 1.0, you might want to generate random numbers within a specific range. You can achieve this by multiplying the output of Math.random() by the size of the range, then adding the start value of the range.
Here’s an example of generating a random number between 10 and 50:
double random = 10 + (Math.random() * 40);
System.out.println(random);
# Output:
# A random number between 10.0 (inclusive) and 50.0 (exclusive)
In this code, Math.random() * 40
generates a random number between 0.0 and 40.0. By adding 10 to this value, we shift the range to 10.0 – 50.0.
Generating Random Integers in Java
If you need to generate random integers instead of doubles, you can use Math.random() in combination with type casting.
Here’s an example of generating a random integer between 0 and 5:
int random = (int) (Math.random() * 6);
System.out.println(random);
# Output:
# A random integer between 0 (inclusive) and 5 (inclusive)
In this code, (int) (Math.random() * 6)
generates a random double between 0.0 and 6.0 and then casts it to an integer. The casting operation truncates the decimal part, resulting in an integer. Note that because the upper limit (6.0) is exclusive, the highest integer we can get is 5.
Exploring Alternative Methods for Random Number Generation in Java
While Math.random() is a simple and effective way to generate random numbers in Java, there are other methods that offer more flexibility and control. Let’s explore two of them: the Random class and the ThreadLocalRandom class.
Using the Random Class in Java
The Random class in Java is used to generate pseudo-random numbers. Unlike Math.random(), the Random class can generate both integer and floating-point numbers, and it allows you to specify a range.
Here’s an example of using the Random class to generate a random integer between 0 and 5:
import java.util.Random;
Random rand = new Random();
int random = rand.nextInt(6);
System.out.println(random);
# Output:
# A random integer between 0 (inclusive) and 5 (inclusive)
In this code, we first import the Random class. We then create a new Random object and use its nextInt method to generate a random integer. The argument passed to nextInt specifies the upper limit of the range (exclusive).
Using the ThreadLocalRandom Class in Java
The ThreadLocalRandom class in Java is another option for generating random numbers. It’s especially useful in multi-threaded environments, as it reduces contention and overhead associated with Random.
Here’s an example of using ThreadLocalRandom to generate a random integer between 0 and 5:
import java.util.concurrent.ThreadLocalRandom;
int random = ThreadLocalRandom.current().nextInt(6);
System.out.println(random);
# Output:
# A random integer between 0 (inclusive) and 5 (inclusive)
In this code, we first import the ThreadLocalRandom class. We then use the current method to get the current thread’s ThreadLocalRandom, and call its nextInt method to generate a random integer.
While these methods offer more functionality than Math.random(), they also require more understanding of Java’s classes and methods. As always, the best approach depends on your specific needs and the context of your project.
Troubleshooting Common Issues with Math.random() in Java
While Math.random() is a powerful tool for generating random numbers in Java, it’s not without its challenges. Here, we’ll discuss some common issues you might encounter and how to resolve them.
Generating Numbers Within a Certain Range
A common issue when using Math.random() is generating numbers within a certain range. As we’ve discussed, Math.random() generates a double between 0.0 (inclusive) and 1.0 (exclusive). If you need a number within a different range, you’ll need to adjust the output.
Here’s how you can generate a number between 10 and 20:
double random = 10 + (Math.random() * 10);
System.out.println(random);
# Output:
# A random number between 10.0 (inclusive) and 20.0 (exclusive)
In this code, Math.random() * 10
generates a number between 0.0 and 10.0. Adding 10 shifts the range to 10.0 – 20.0.
Generating Random Integers
Another common challenge is generating random integers. Math.random() generates doubles, not integers. To get an integer, you’ll need to cast the double to an int. This removes the decimal part.
Here’s how you can generate an integer between 0 and 5:
int random = (int) (Math.random() * 6);
System.out.println(random);
# Output:
# A random integer between 0 (inclusive) and 5 (inclusive)
In this code, (int) (Math.random() * 6)
generates a double between 0.0 and 6.0 and casts it to an int, removing the decimal part. Note that the upper limit (6.0) is exclusive, so the highest possible integer is 5.
Best Practices and Optimization
When using Math.random(), keep these best practices in mind:
- Understand the output. Math.random() generates a double between 0.0 (inclusive) and 1.0 (exclusive). Make sure this is what you need for your program.
- Use casting for integers. If you need an integer, cast the double to an int.
- Adjust the range as needed. If you need a number within a certain range, adjust the output of Math.random() accordingly.
- Consider alternative methods. If Math.random() doesn’t meet your needs, consider using the Random or ThreadLocalRandom classes.
Understanding Random Number Generation in Java
To fully grasp how Math.random() works in Java, it’s essential to delve into the concept of random number generation. In the world of computers and programming, the term ‘random’ often refers to something known as ‘pseudorandom’.
The Concept of Pseudorandom Numbers
Pseudorandom numbers are sequences of numbers that appear random, but are generated by a deterministic process. This means that given the same initial state (known as the seed), the sequence of numbers will be the same every time. This is in contrast to true random numbers, which are completely unpredictable and do not follow any deterministic process.
How Math.random() Generates Pseudorandom Numbers
Math.random() in Java generates pseudorandom numbers using a specific algorithm. The function doesn’t require any arguments and returns a double value that is greater than or equal to 0.0 and less than 1.0. Each time you call Math.random(), it generates the next number in its pseudorandom sequence.
Here’s a simple example:
double random = Math.random();
System.out.println(random);
# Output:
# A pseudorandom double between 0.0 (inclusive) and 1.0 (exclusive)
In this code, Math.random()
generates a pseudorandom number and assigns it to the random
variable. The System.out.println(random);
line then prints this pseudorandom number to the console.
By understanding the theory behind random number generation and the concept of pseudorandom numbers, you can better understand how Math.random() works and how to use it effectively in your Java programs.
Exploring the Impact of Random Number Generation
Random number generation, such as with Math.random() in Java, plays a crucial role in many larger projects. It’s not just about creating a dice roll or picking a random element from an array. The applications are vast and varied, from game development to simulations and beyond.
Random Number Generation in Game Development
In game development, random number generation can be used to create unpredictable environments, spawn enemies, randomize loot, and much more. This unpredictability can make a game more challenging and replayable, as each playthrough offers a unique experience.
Simulations and Random Number Generation
Simulations often rely on random number generation to mimic the randomness inherent in real-world phenomena. For example, a weather simulation might use random numbers to represent the probability of various weather events.
Using Random Numbers in Algorithms
Many algorithms, especially in the field of machine learning, use random numbers. For example, random numbers can be used to initialize weights in neural networks, or to split a dataset into training and testing sets.
Generating Random Strings in Java
Random number generation can also be used to create random strings in Java, which can be useful for generating passwords, tokens, or identifiers.
Here’s a simple example of generating a random string:
import java.util.UUID;
String randomString = UUID.randomUUID().toString();
System.out.println(randomString);
# Output:
# A random UUID, such as '3b241101-e2bb-4255-8caf-4136c566a964'
In this code, UUID.randomUUID().toString()
generates a random UUID and converts it to a string. The System.out.println(randomString);
line then prints this random string to the console.
Further Resources for Mastering Randomness in Java
To deepen your understanding of random number generation in Java and its applications, you might find the following resources helpful:
- Beginner’s Guide to Using Math Class in Java – Discover essential methods in Java’s Math class for arithmetic operations.
Exploring Math.pow() Function in Java – Learn how Math.pow() simplifies raising base number to a specified power in Java.
Absolute Value in Java – Learn how to compute the absolute value of a number using Math.abs() in Java.
Java: Generating Random Numbers – Learn to generate random numbers in Java with this guide from GeeksforGeeks.
Java Random Number Generation – Tutorialspoint’s tutorial on the mechanisms for generating random numbers in Java.
Java Random Class – Dive into Java’s Random class methods with Javatpoint’s tutorial.
These resources offer in-depth explanations and examples that can help you master the use of Math.random() and other methods of generating random numbers in Java.
Wrapping Up: Mastering Math.random() in Java
In this comprehensive guide, we’ve demystified the use of Math.random() in Java, a powerful tool for generating random numbers.
We began with the basics, explaining how to use Math.random() to generate random double values between 0.0 and 1.0. We then advanced to generating random numbers within a specific range and even generating random integers. We discussed the potential pitfalls and how to navigate them, providing practical code examples along the way.
We didn’t stop at Math.random(). We also explored alternative methods for generating random numbers in Java, such as the Random class and the ThreadLocalRandom class. Each of these methods has its own advantages and use-cases, and understanding them gives you more tools in your Java programming toolbox.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Math.random() | Simple, easy to use | Only generates doubles, limited range |
Random class | Generates both doubles and integers, can specify range | Requires creating a Random object |
ThreadLocalRandom class | Ideal for multi-threaded environments, generates both doubles and integers, can specify range | Requires more understanding of Java’s classes and methods |
Whether you’re a Java beginner or an experienced developer looking to refresh your knowledge, we hope this guide has provided you with a deeper understanding of random number generation in Java.
With the ability to generate random numbers, you can add unpredictability to your programs, simulate real-world phenomena, and solve complex problems. Now, you’re well equipped to harness the power of randomness in your Java projects. Happy coding!